Usage
Installation
To use fpdf-table, first install it from PyPi using pip:
(.venv) $ pip install fpdf-table
Minimal Example
Code
from fpdf_table import PDFTable
def minimal_example():
data: list[list[str]] = [
['Gerard', 'Martinez', '09/07/1998'],
['Amy ', 'Miller', 'July 30, 1969'],
['Ferdinand ', 'Varela ', 'November 10, 1988'],
['Edén ', 'Mascarenas Benavides', 'May 23, 1990'],
['Adrián ', 'Beltrán ', 'December 12, 1977'],
]
# initialize PDFTable, before doing anything, __init__ adds a page, sets font, size and colors
pdf = PDFTable()
# table header
pdf.table_header(['First Name', 'Last Name', 'Date of birth'])
# table rows
for person in data:
pdf.table_row(person)
# file path where to save the pdf
pdf.output("../pdfs/minimal_example.pdf")
minimal_example()
PDF
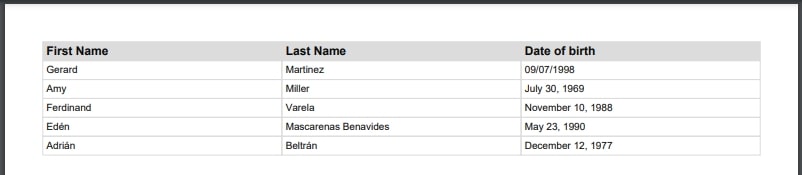
Main Features
Code
from fpdf_table import PDFTable, Align
def features_example():
# initialize PDFTable, before doing anything, __init__ adds a page, sets font, size and colors
pdf = PDFTable()
"""
table row
"""
# draw a table header, pass a list with the text, by default width is the same for every column
# and align is to left
pdf.table_header(['First Name', 'Last Name', 'Date of birth'])
# draw a table row, by default is only one row with height equal to pdf.default_cell_height
pdf.table_row(['Gerard', 'Martinez', '09/07/1998'])
"""
responsive row
"""
# header with custom width
pdf.table_header(['Email', 'Address'], [pdf.calculate_width_3(), 2 * pdf.calculate_width_3()])
# responsive row with custom width
pdf.table_row(['large_email_example-very_large_email_example-more_large_email_example@example.com',
'952 Rogers Ave, Okanogan, Washington(WA), 98840'],
pdf.table_cols(4, 8), option='responsive')
"""
fixed height row
"""
# align center, expects a list of alignments but if you pass only one it spreads for every column
pdf.table_header(['Description'], align=Align.C)
large_text = """Lorem Ipsum is simply dummy text of the printing and typesetting industry....."""
# fixed row needs fixed_height parameter
pdf.table_row([large_text], option='fixed', fixed_height=6 * pdf.row_height_cell)
# output
pdf.output("../pdfs/main_features.pdf")
features_example()
PDF
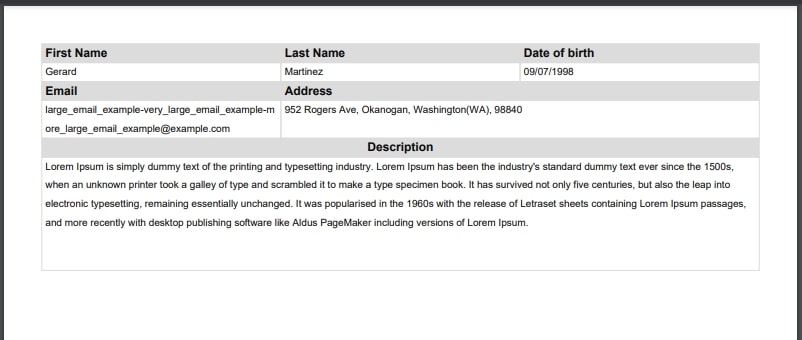
Image Features
Code
from fpdf_table import PDFTable, add_image_local
def image_example():
# initialize PDFTable, before doing anything, __init__ adds a page, sets font, size and colors
pdf = PDFTable()
# load image from file
img, img_width, img_height = add_image_local('../pdfs/logo1.png')
# set custom width and height
img_width, img_height = pdf.use_px_to_mm(150), pdf.use_px_to_mm(150)
# draw image, center on page
pdf.draw_image_center(img=img, img_width=img_width, img_height=img_height, container_width=pdf.get_width_effective())
# line breaks
pdf.ln(img_height)
pdf.ln(10)
# get cursor position
x, y = pdf.get_x(), pdf.get_y()
# draw a fixed table without content
table_height = pdf.use_px_to_mm(200)
# change color of table border
pdf.set_draw_color(10, 10, 10)
pdf.table_row(['', '', ''], option='fixed', fixed_height=table_height)
# draw image no align
pdf.draw_image_center(img=img, x=x, y=y, img_width=img_width, img_height=img_height)
# draw image center horizontally
x = x + pdf.calculate_width_3()
pdf.draw_image_center(img=img, x=x, y=y, img_width=pdf.use_px_to_mm(150), img_height=pdf.use_px_to_mm(150),
container_width=pdf.calculate_width_3())
# draw image center horizontally and vertically
x = x + pdf.calculate_width_3()
pdf.draw_image_center(img=img, x=x, y=y, img_width=pdf.use_px_to_mm(150), img_height=pdf.use_px_to_mm(150),
container_width=pdf.calculate_width_3(), container_height=table_height)
# file path where to save the pdf
pdf.output("../pdfs/image_example.pdf")
image_example()
PDF
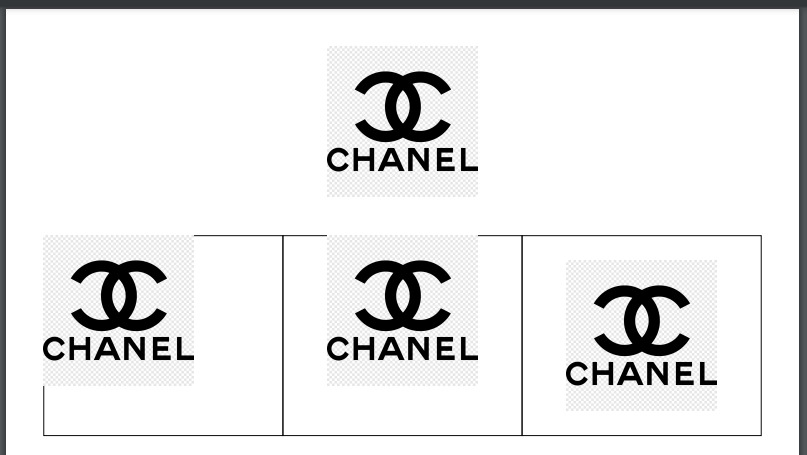
Usage in web APIs
Please refer to fpdf2 usage in web APIs